Navigation between screens using React native
1. Add Navigation Package
To navigate between apps multiple screen, react native provides a react-navigation package.
React Navigation provides an easy to use navigation solution.It has two navigation options. stack navigation and tabbed navigation, which supports in both iOS and Android.
yarn add react-navigation
2. Stack Navigation Example
Stack Navigator accept object as an argument where each screen specified by a key value pair which returns a React Component. Using those Key values only we can navigate through screens. Ex. Home, Profile.
app.js
import React from 'react';
import { View, Text } from 'react-native';
import { StackNavigator } from 'react-navigation';
class HomeScreen extends React.Component {
render() {
return (
<View style={{ flex: 1, alignItems: 'center', justifyContent: 'center' }}>
<Text>Home Screen</Text>
</View>
);
}
}
class DetailsScreen extends React.Component {
render() {
return (
<View style={{ flex: 1, alignItems: 'center', justifyContent: 'center' }}>
<Text>Details Screen</Text>
</View>
);
}
}
const RootStack = StackNavigator({
Home: {
screen: HomeScreen,
},
Details: {
screen: DetailsScreen,
}
},
{
initialRouteName: 'Home',
});
export default class App extends React.Component {
render() {
return <RootStack />;
}
}
If you run this code, you will see a screen with an empty navigation bar and a grey content area containing your HomeScreen component.
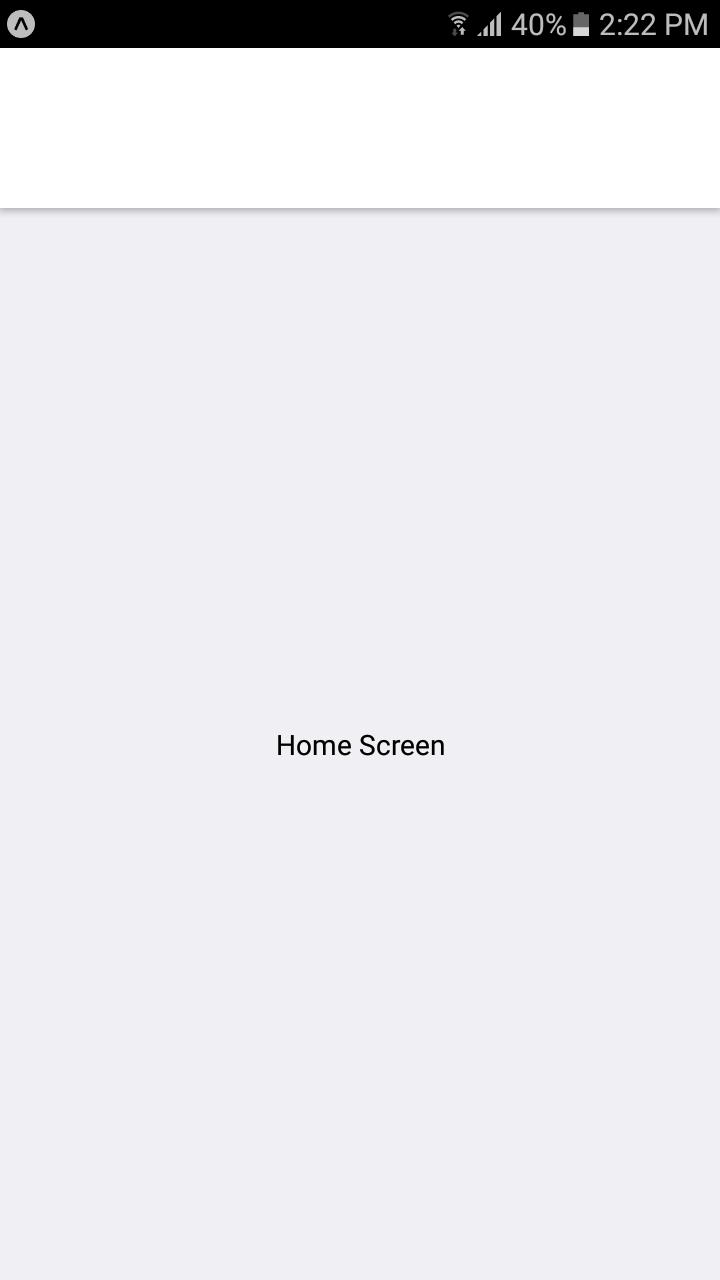
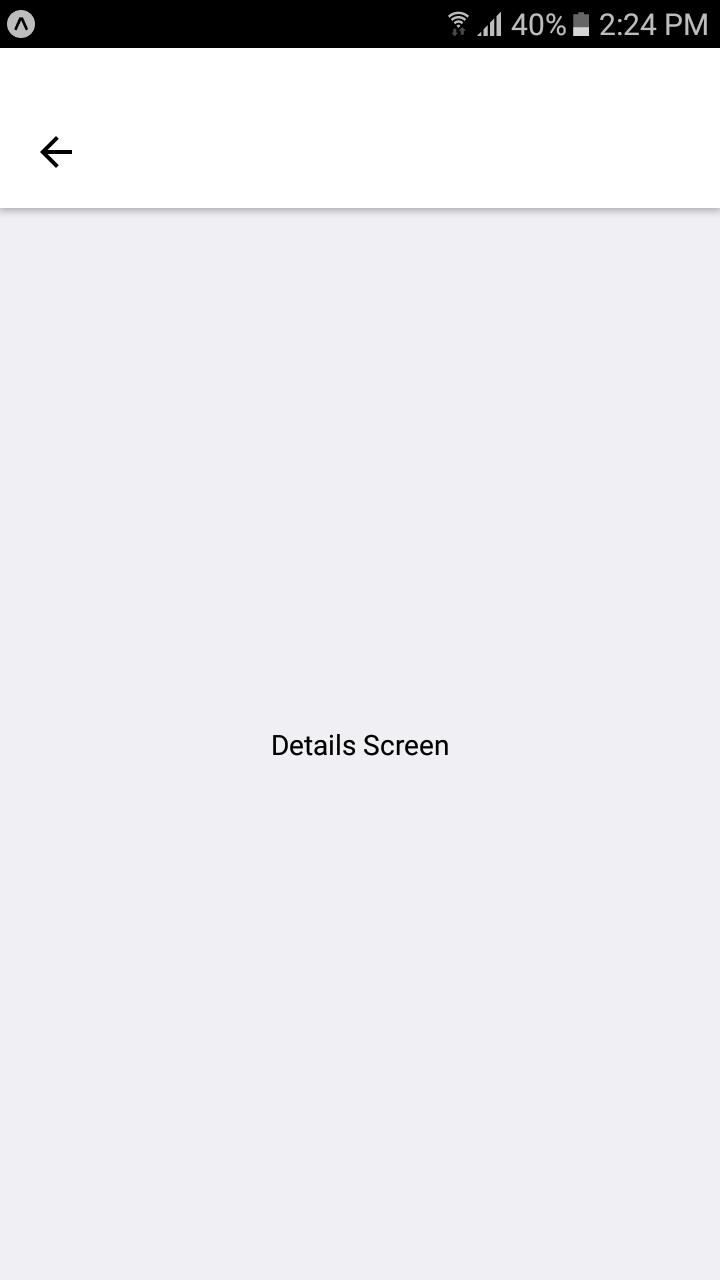
To specify what the initial route in a stack is, provide an initialRouteName on the stack options object.
3. Navigating to a new screen? How?
import React from 'react';
import { Button, View, Text } from 'react-native';
import { StackNavigator } from 'react-navigation';
class HomeScreen extends React.Component {
render() {
return (
<View style={{ flex: 1, alignItems: 'center', justifyContent: 'center' }}>
<Text>Home Screen</Text>
<Button
title="Go to Details"
onPress={() => this.props.navigation.navigate('Details')}
/>
</View>
);
}
}
// ... other code from the previous section
this.props.navigation.navigate() is a method used to navigate from "Home screen" to "Details screen".This method injected to each routes which defined in StackNavigator.
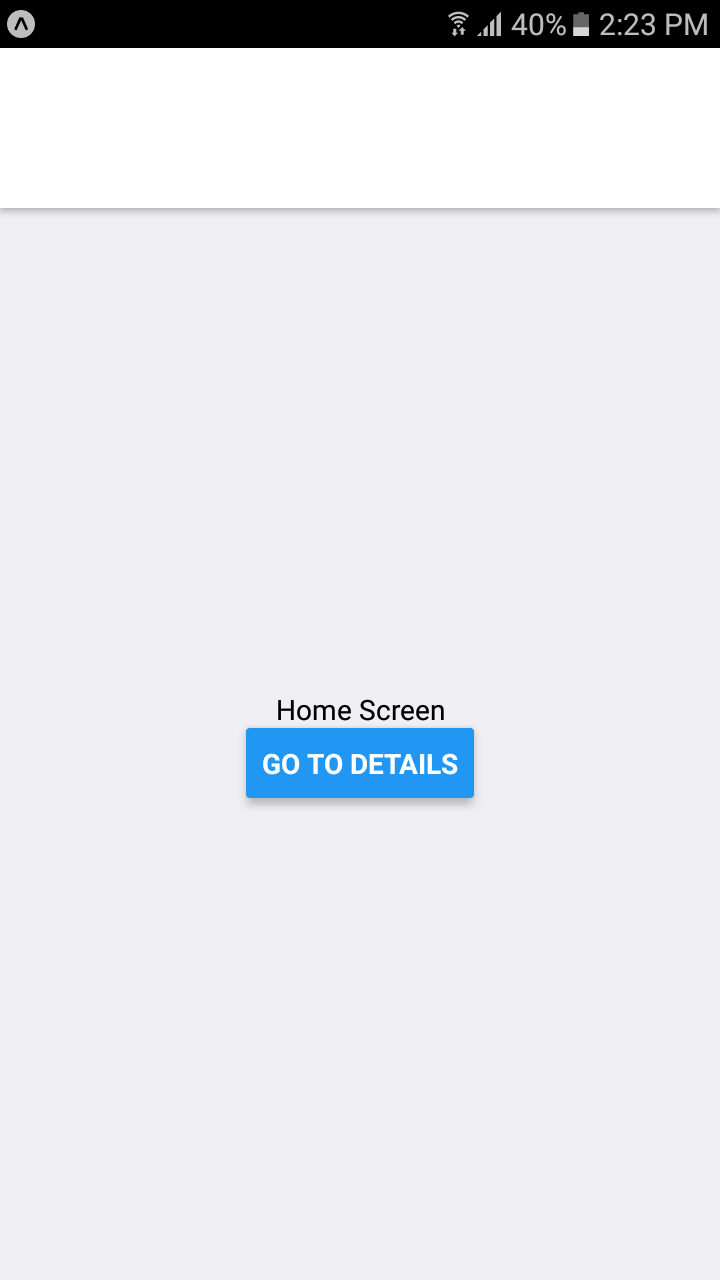
4. How to navigate back?
// ... other code from the previous section
class DetailsScreen extends React.Component {
render() {
return (
<View style={{ flex: 1, alignItems: 'center', justifyContent: 'center' }}>
<Text>Details Screen</Text>
<Button
title="Go to Details... again"
onPress={() => this.props.navigation.navigate('Details')}
/>
<Button
title="Go back"
onPress={() => this.props.navigation.goBack()}
/>
</View>
);
}
}
// ... other code from the previous section
The header bar will automatically show a back button.But you can programmatically go back by calling this method this.props.navigation.goBack().
Whenever you click the Go to Details... again button it pushes a new route to the navigation stack.
- Stack navigator header has it's own back button like left arrow.
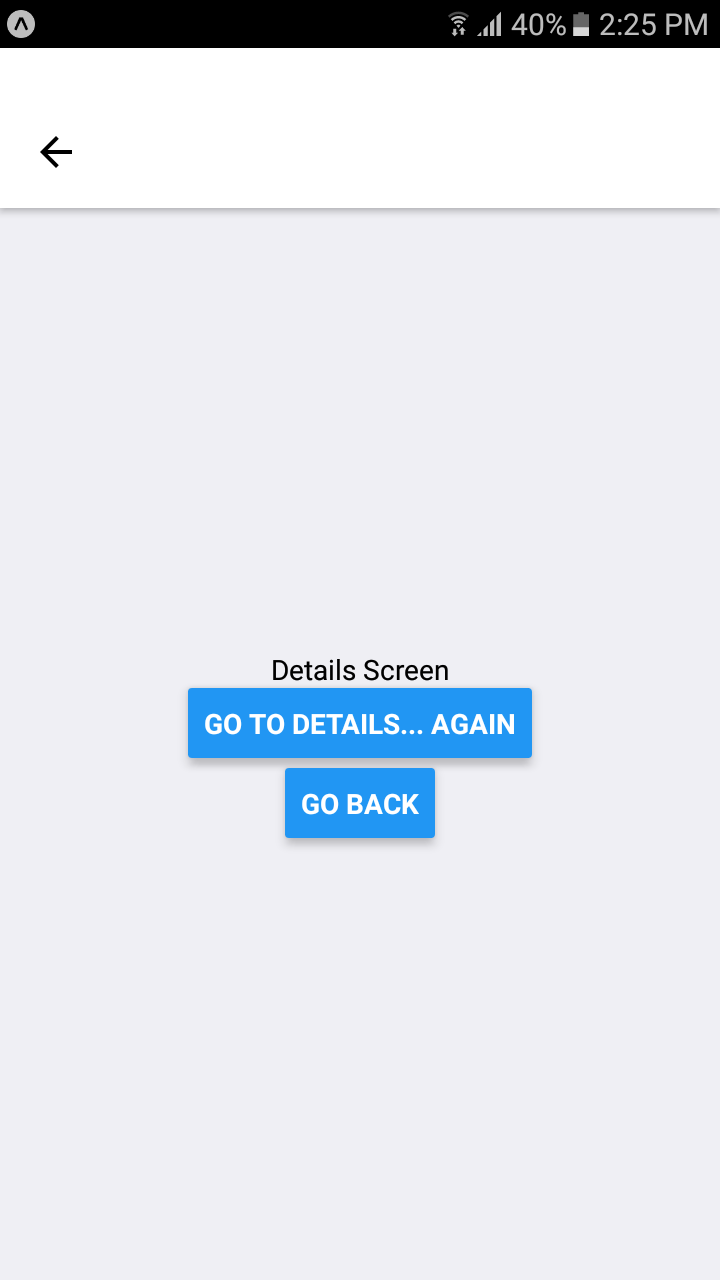
5. How to configure the Header bar in a screen?
class HomeScreen extends React.Component {
static navigationOptions = {
title: 'Home',
};
/* render function, etc */
}
class DetailsScreen extends React.Component {
static navigationOptions = {
title: 'Details',
};
/* render function, etc */
}
The screens which are used in Stack Navigator can have a static property called navigationOptions. It accepts object or function as an argument and returns an object which contains various configurations.
Here i am changing the title of each screen by setting navigationOptions title.
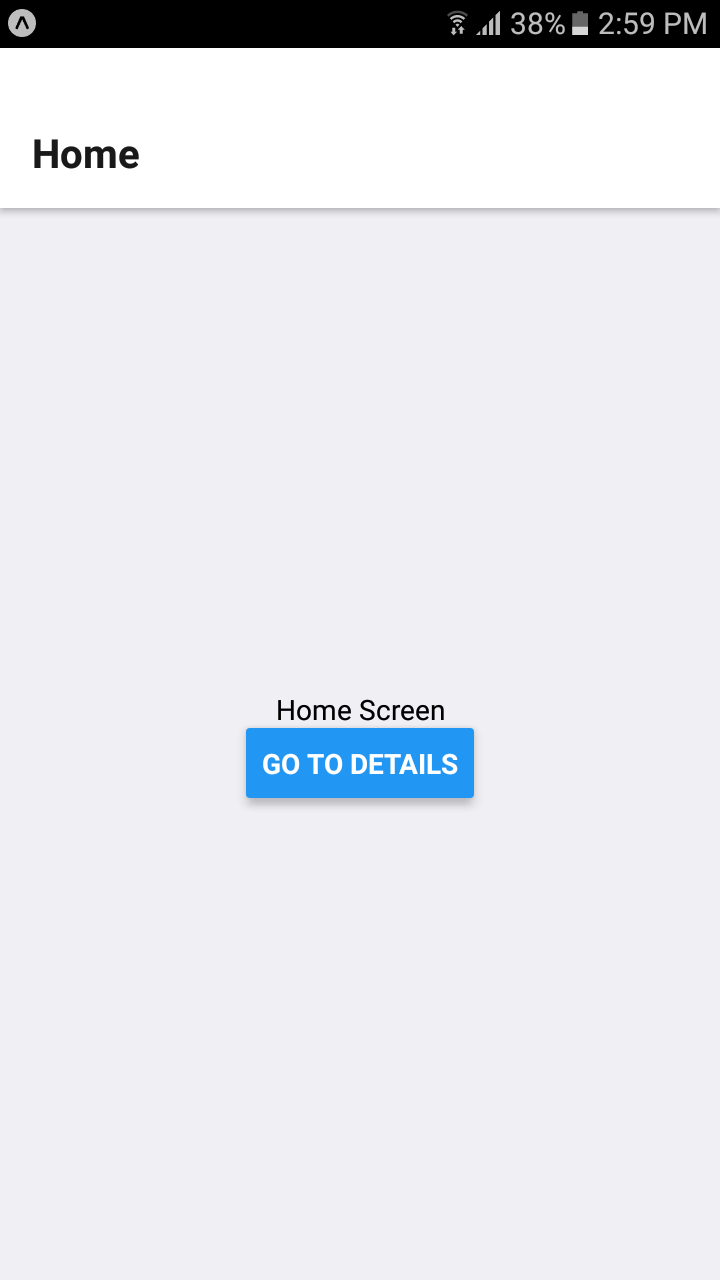
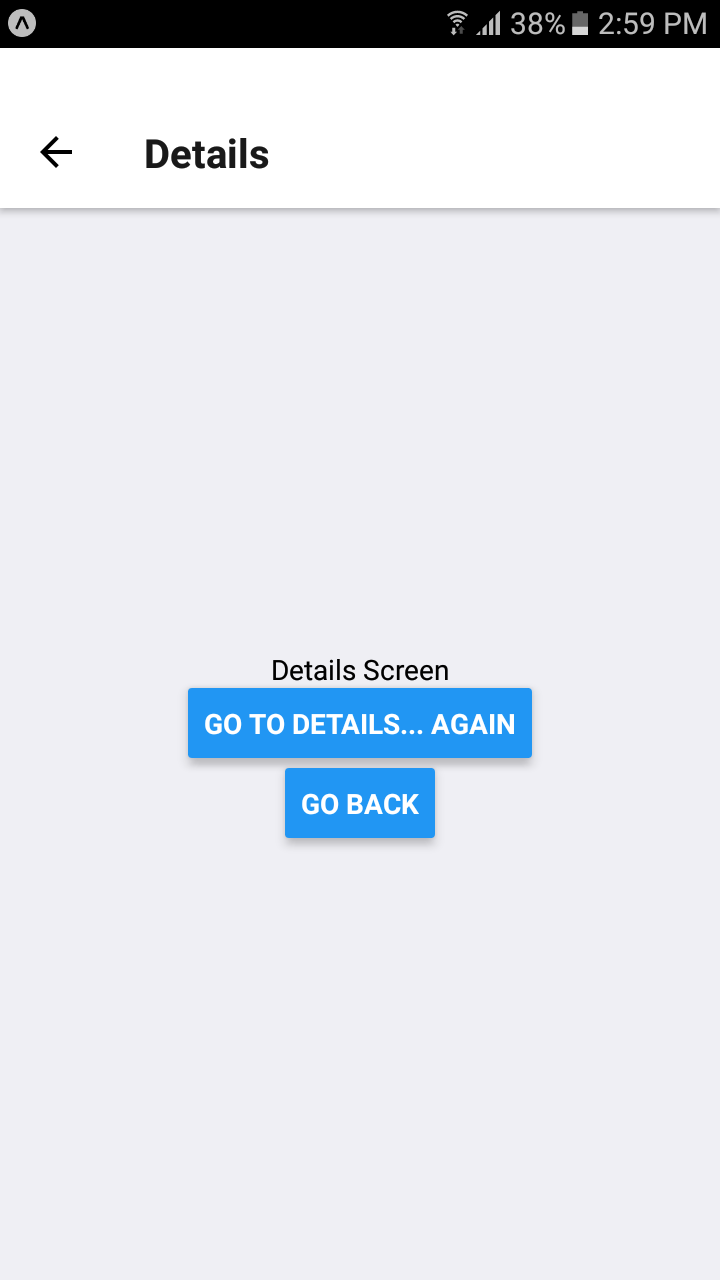
5. How to share a commmon navigationOptions in all screens?
class HomeScreen extends React.Component {
static navigationOptions = {
title: 'Home',
/* No more header config here! */
};
/* render function, etc */
}
/* other code... */
const RootStack = StackNavigator(
{
Home: {
screen: HomeScreen,
},
Details: {
screen: DetailsScreen,
},
},
{
initialRouteName: 'Home',
/* The header config from HomeScreen is now here */
navigationOptions: {
headerStyle: {
backgroundColor: '#f4511e',
},
headerTintColor: '#fff',
headerTitleStyle: {
fontWeight: 'bold',
},
},
}
);
Here i am writing a common navigationOptions configurations in a Stack navigator which applies to all the screen defined in stack navigator.
For Ex. headerStyle, headerTintColor, headerTitleStyle.
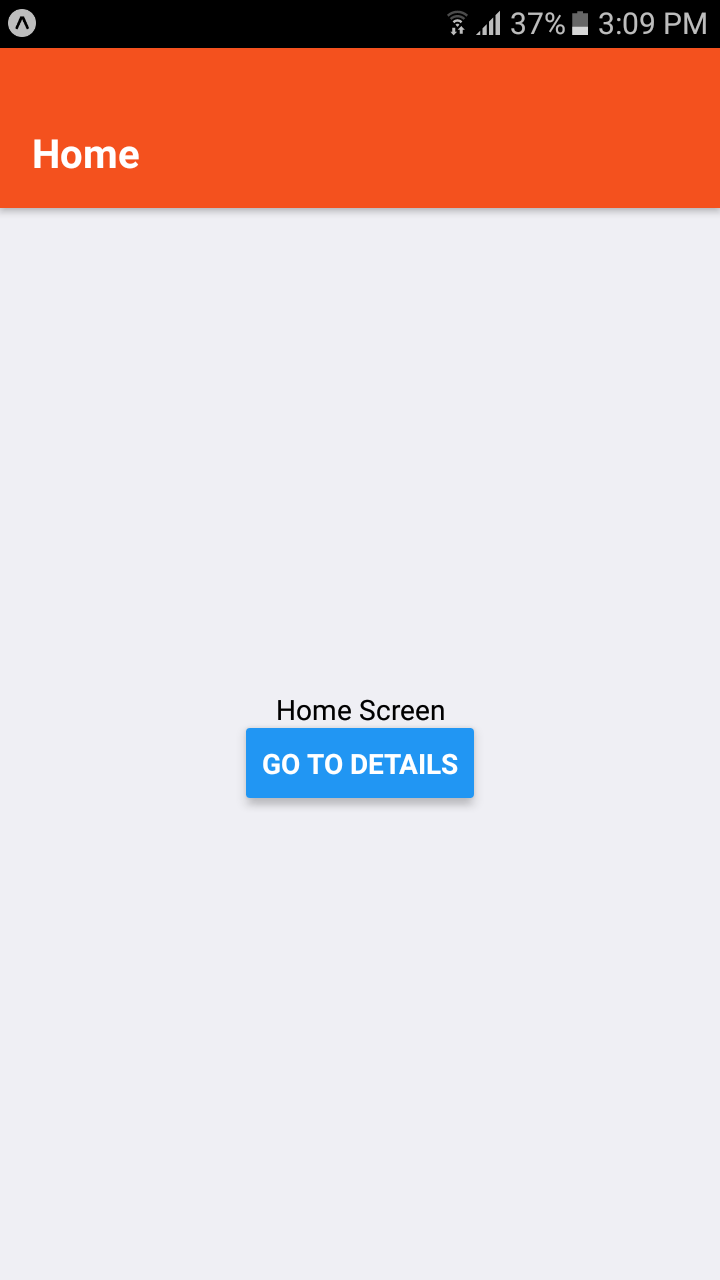
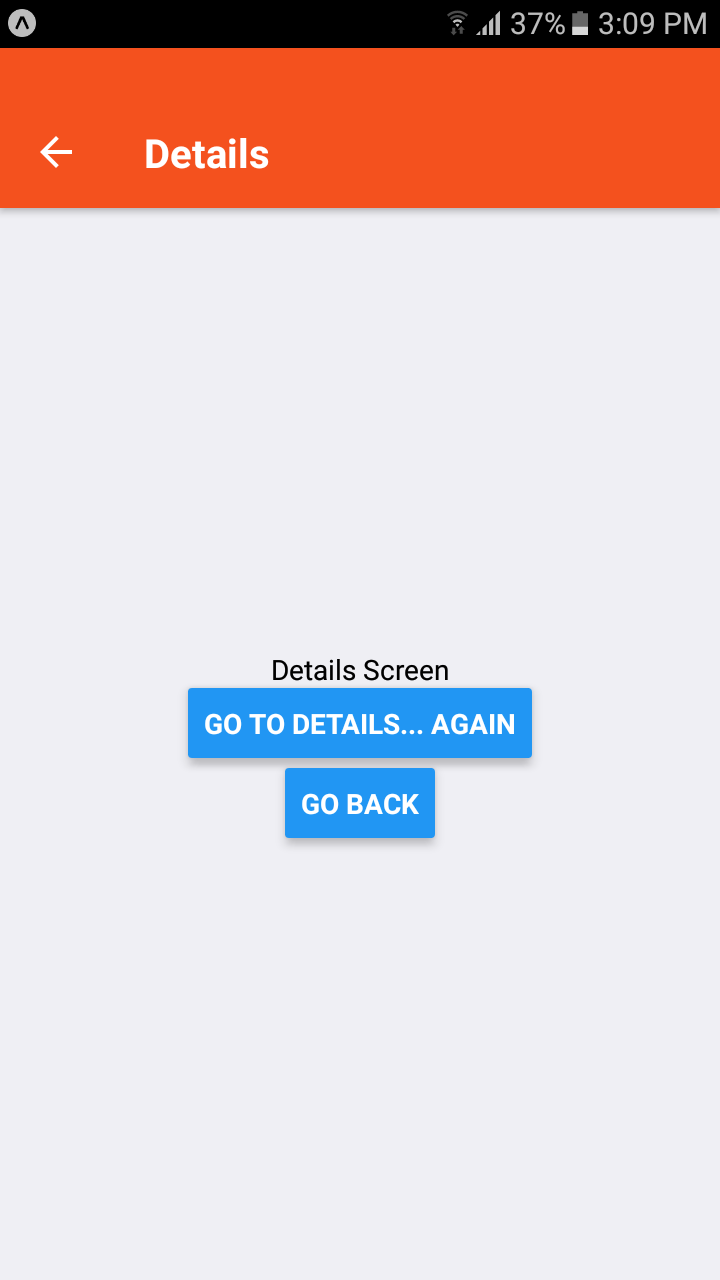