Login page using React Native
User login and authentication is a primary functionality. Let's see how to create a Login Page and authenticate user.
1. Creat a component
To create a login page we need an input components like Input boxes to enter username and password and Button component to submit values and invoke authentication functions.
import React, { Component } from "react";
import { View, TextInput, Button } from "react-native";
class LoginScreen extends Component{
state = {
phone: "",
password: "",
message:""
};
login=()=>{
}
render(){
return <View>
<TextInputplaceholder="Phone" style={styles.input} onChangeText={e => this.setState({ phone: e })}/>
<TextInputplaceholder="Password" style={styles.input} onChangeText={e => this.setState({ password: e })}/>
<Text style={styles.messagetext}>{this.state.message}</Text>
<Button onPress={this.login}>Login</Button>
</View>
}
}
//Write your own css style here.
const styles = StyleSheet.create({....});
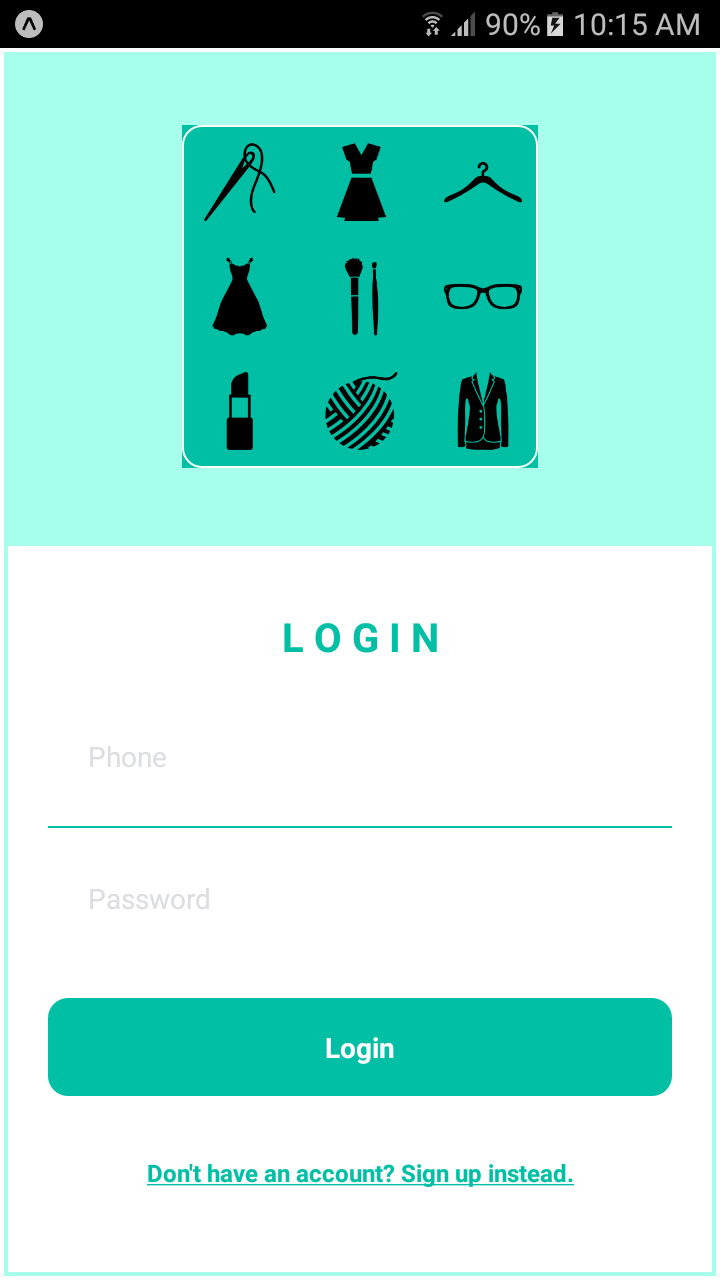
2. Define a login function
Here i am gonna do 3 important things.
1. Connect to a database.
2. Fetch Result
3. Process Result
The above three process done by using react native fetch method.It is same as browser fetch API.
login=()=>{
const {phone,password} = this.state;
const query= {
//Write your query
};
const DB_ENDPOINT ="https://your-database-api-endpoint";
const options = {
uri: DB_ENDPOINT,
headers: { 'Content-Type': 'application/json' },
method: 'POST',
body: JSON.stringify({ query })
};
fetch(DB_ENDPOINT, options).then(resp => {
return resp.json()
}).then(res => {
//Returned result maybe different for you.
if (res.data.result) {
this.setState({ message: "Successfully logged in" });
} else {
this.setState({ message: "Invalid credentials" });
}
return res;
}) .catch(() => {
this.setState({ message: "Invalid credentials" });
});
}
3. Display result
Now we have to enter a phone number and password to login.The Authenticaiton result will be displayed in message text and then we need to navigate if the result is success.
Previous
Next